Here we going to learn about cache implementation in Web Applications. I have an application in ASP.Net with Self Host WCF Services.
Why do we need a cache?
We are facing many issues related to application performance. To overcome this, we can use Cache to avoid multiple calls to DB. We store the data in a Cache at 1st call, afterward when you are about to fetch the data it will be retrieved from the Cache. Also, we set cache expiry duration too. If the cache is not available, then we load the data into the cache again. Do not store huge data in the cache, again it leads to performance issues, we have several concepts to store huge data in memory.
Let’s jump into the implementation directly.
Add System.Runtime.Caching library into your application.
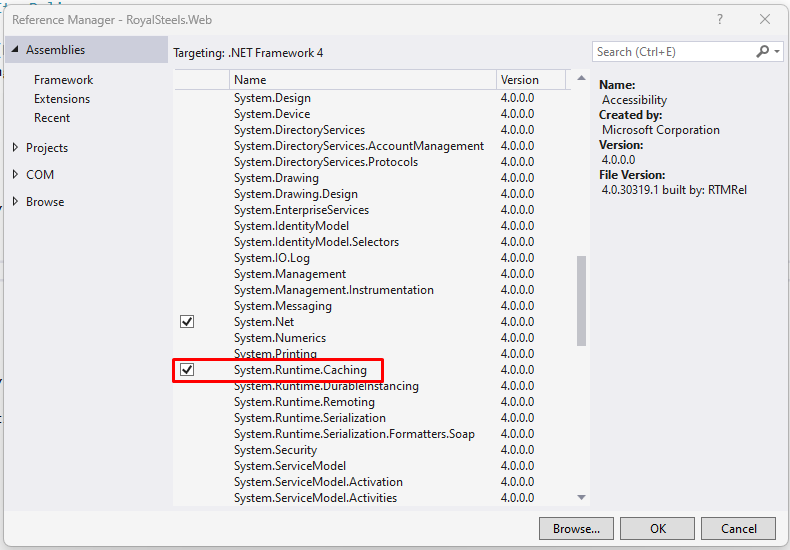
Declare the Cache in WCF Services
using System;
using System.Web;
using System.Web.Script.Serialization;
using System.Collections.ObjectModel;
using System.Globalization;
using System.Runtime.Caching;
public partial class RoyalSteelsService : IRoyalSteelsService
{
ObjectCache cache = MemoryCache.Default;
CacheItemPolicy cacheItemPolicy = new CacheItemPolicy
{
AbsoluteExpiration = new DateTimeOffset(DateTime.UtcNow.AddMinutes(5)),
SlidingExpiration = ObjectCache.NoSlidingExpiration,
};
C#Example: We take the example of Manage Tax Page
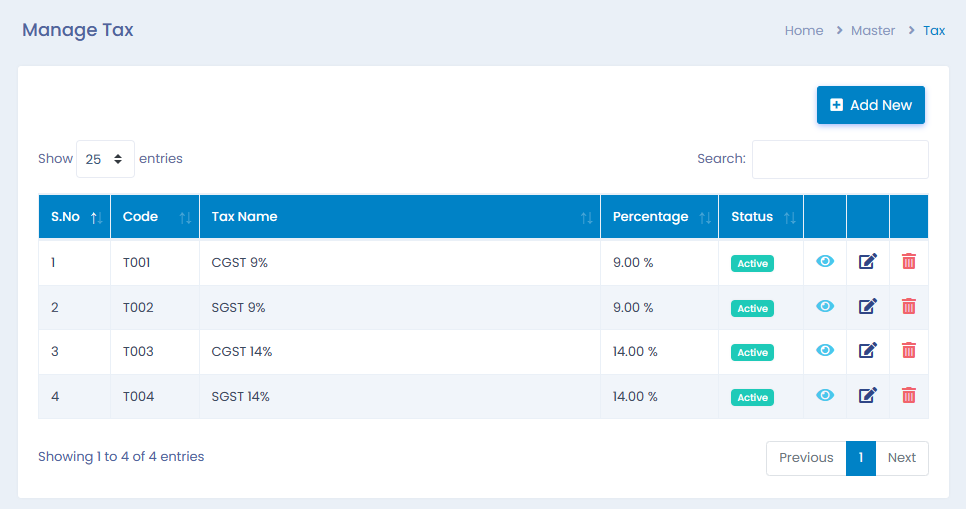
Code Block of Manage Tax in Service
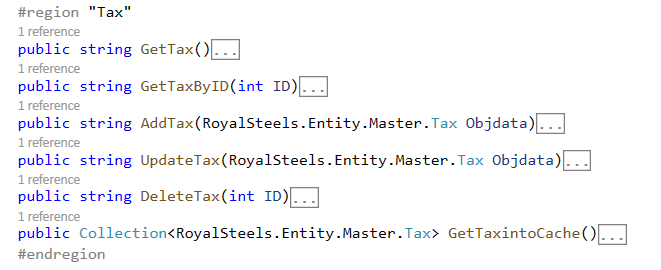
Code for loading the Tax Data into Cache
public Collection<RoyalSteels.Entity.Master.Tax> GetTaxintoCache()
{
Collection<RoyalSteels.Entity.Master.Tax> ObjList = new Collection<RoyalSteels.Entity.Master.Tax>();
if (cache["_cacheTaxData"] != null)
{
ObjList = (Collection<RoyalSteels.Entity.Master.Tax>)cache.Get("_cacheTaxData");
}
else
{
ObjList = RoyalSteels.DataAccess.Master.TaxDAL.GetTax();
cache.Add("_cacheTaxData", ObjList, cacheItemPolicy);
}
return ObjList;
}
C#How can we reuse the cache in the listing?
public string GetTax()
{
string sException = string.Empty;
string sFileNames = string.Empty;
JavaScriptSerializer jsObject = new JavaScriptSerializer(); jsObject.MaxJsonLength = Int32.MaxValue;
RoyalSteels.Entity.Response objResponse = new RoyalSteels.Entity.Response();
try
{
if (ValidateSession())
{
Collection<RoyalSteels.Entity.Master.Tax> ObjList = new Collection<RoyalSteels.Entity.Master.Tax>();
if (cache["_cacheTaxData"] != null)
objResponse.ADValueI = "Data from Cache";
else
objResponse.ADValueI = "Data from DB";
ObjList = GetTaxintoCache();
objResponse.Status = "Success";
objResponse.Value = ObjList.Count > 0 ? jsObject.Serialize(ObjList) : "NoRecord";
}
else
{
objResponse.Status = "Error";
objResponse.Value = "0";
}
}
catch (Exception ex)
{
sException = "RoyalSteelsService.GetTax() |" + ex.Message.ToString();
Log.Write(sException);
objResponse.Status = "Error";
objResponse.Value = "Error";
}
return jsObject.Serialize(objResponse);
}
C#Load/Reset the cache again when Add/Update/Delete happens. In-Line No 20, we reset the cache to Null. GetTax() Method is called to load the data after Add/Update/Delete happens. I have used the same line (Line 20) in AddTax, UpdateTax after the successful call from DB.
public string DeleteTax(int ID)
{
string sTaxId = string.Empty;
string sException = string.Empty;
JavaScriptSerializer jsObject = new JavaScriptSerializer(); jsObject.MaxJsonLength = Int32.MaxValue;
RoyalSteels.Entity.Response objResponse = new RoyalSteels.Entity.Response();
bool bTax = false;
int UserId = 0;
try
{
if (ValidateSession())
{
UserId = Convert.ToInt32(((RoyalSteels.Entity.UserManagement.User)HttpContext.Current.Session["RoyalUserDetails"]).UserID);
bTax = RoyalSteels.DataAccess.Master.TaxDAL.DeleteTax(ID, UserId);
if (bTax == true)
{
objResponse.Status = "Success";
objResponse.Value = "1";
if (cache["_cacheTaxData"] != null) cache.Remove("_cacheTaxData");
}
else
{
objResponse.Status = "Success";
objResponse.Value = "0";
}
}
else
{
objResponse.Status = "Error";
objResponse.Value = "0";
}
}
catch (Exception ex)
{
sException = "RoyalSteelsService.Tax.DeleteTax() |" + ex.Message.ToString();
Log.Write(sException);
if (ex.Message.ToString() == "Tax_R_01" || ex.Message.ToString() == "Tax_D_01")
{
objResponse.Status = "Error";
objResponse.Value = ex.Message.ToString();
}
else
{
objResponse.Status = "Error";
objResponse.Value = "0";
}
}
return jsObject.Serialize(objResponse);
}
C#Open Manage Tax Page for the first time
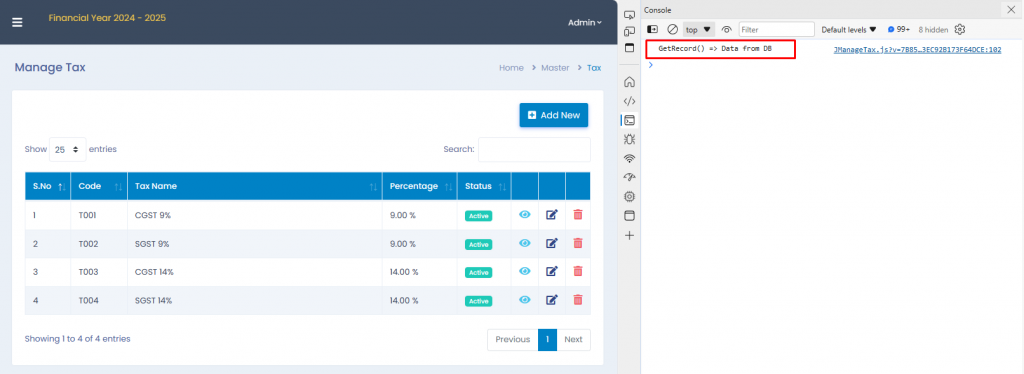
2nd Call
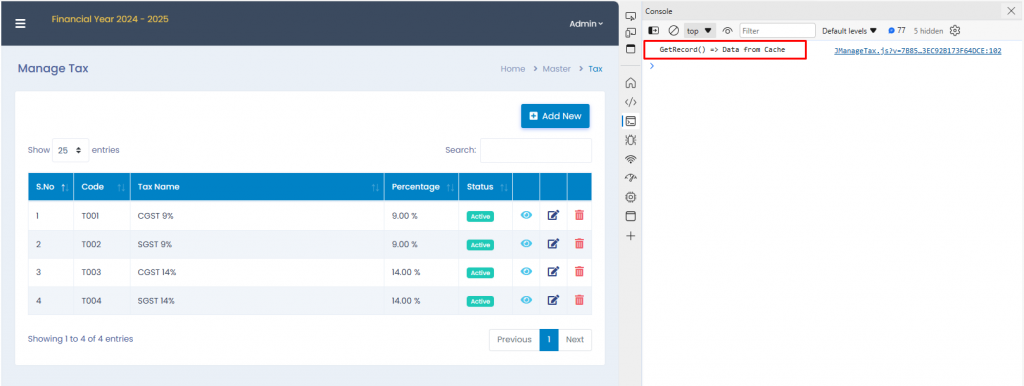
Update call
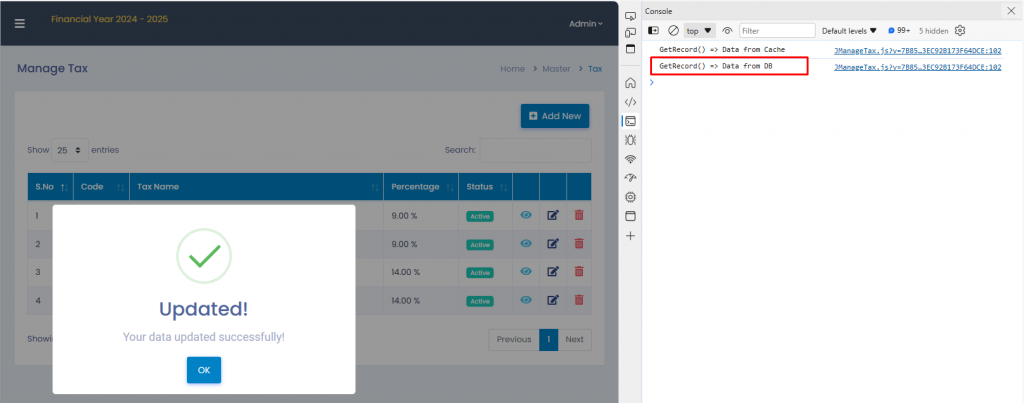